Component 2
2.3 Producing Robust Programs
Defensive design
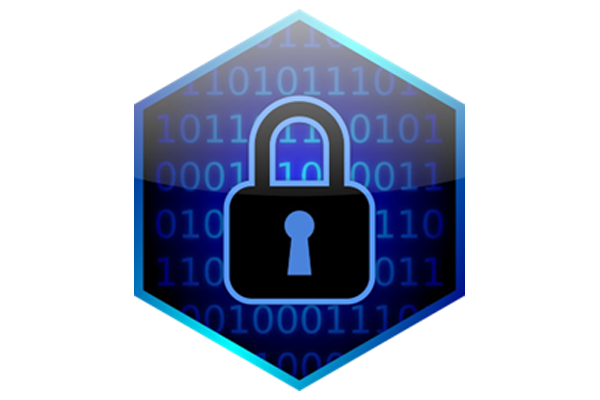
When you design a system you should always assume that the worst will happen. Either somebody will accidentally do something silly to break it or, more likely, a malicious user will deliberately destroy it. It’s important to anticipate misuse and build in safeguards to protect yourself.
Any system that needs to be protected should have suitable authentication routines in place. This is a technical term for the ability to log in.x Authentication will handle usernames and passwords, plus the ability to register, request a password reset link if a password is forgotten and keep track of session times to help to spot maliscious users.
Input validation
Input validation checks to see if a user input is reasonable and within certain parameters. It can't check to see if it is entirely correct but is a useful way of filtering out common mistakes people might make when entering data. Some different types are:
- Length check- checks that an input isn't too long or too short.
- Format check- checks that an input is in the correct format e.g. a postcode or date of birth.
- Presence check- checks that something has been actually entered and it hasn't just been left blank.
- Range check- checks to see if a numerical input is within a certain range.
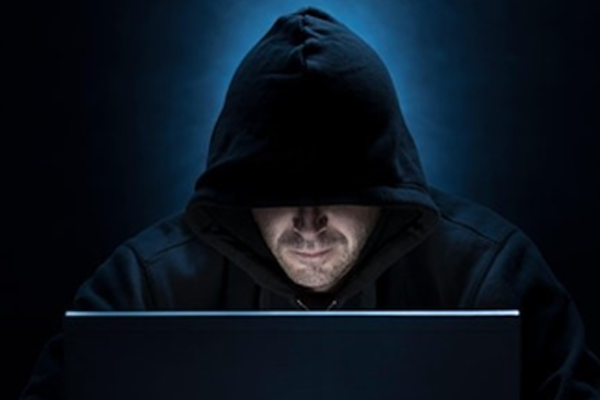
Maintainability
This refers to making your programs easy to debug, easy to work on in the future and easy to work on in a team.
Comments
Adding comments to your code is a sensible way to describe what your code does. This means that when you come back to it later you can remember what it all does. It also helps when other people work on your code.

Indentation
If you indent lines then it makes the code easier to read. Those of you that have learned Python know that this is required to get the code to work! If you have studied another language, you may have an IDE that indents the code for you. Or you may just have to do it yourself!
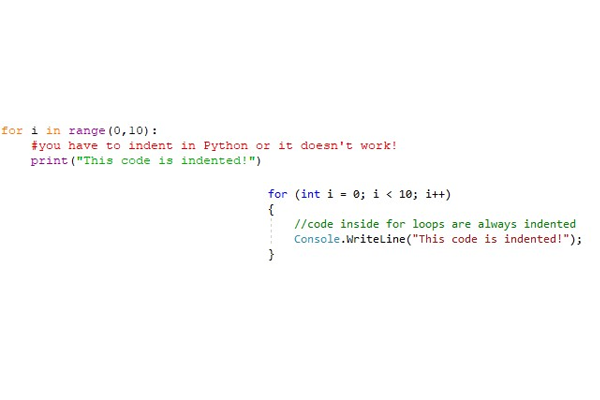
Use of sub-programs
If you split your code up into suitable procedures and functions, it becomes easier to manage and maintain. The name of the sub-programs should reflect what it does. For instance, if your sub-program calculates VAT, then you can call it CalculateVAT() rather than something abstract.
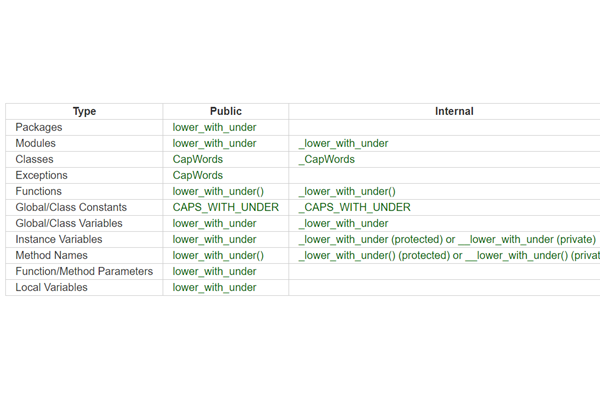
Naming conventions
Following rules for naming variables is a good idea so everyone on the team can easily understand the purpose of each variable.
Testing
Software must be tested to check that it is free from bugs, works as intended and meets the original requirements and success criteria set out in the analysis stage.
There are two main types of testing:
- Iterative- this is testing completed by the development team as the software is being written. They will write tests to ensure the software is functional when each unit is written.
- Final / terminal- this is testing completed once the program has been fully made. Often, it is completed by different people to the development team, possibly in another company. The software will be checked for robustness (ensuring it is error free) and then evaluated against the original requirements to see if everything that should have been included is there.
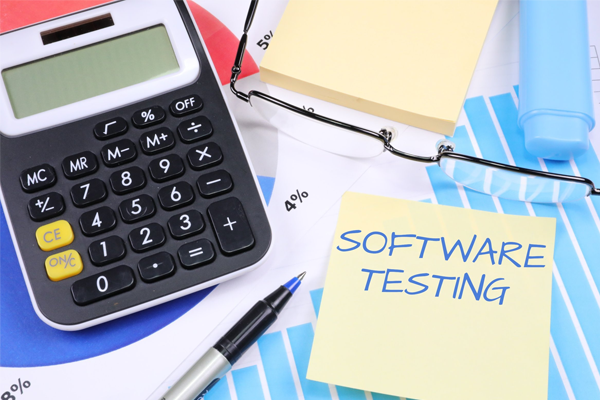
Syntax and logic errors
These are the two main types of error that you can encounter when programming.
- Syntax error- An error when the rules of the language have been broken. This could be anything from a misspelled work or a comma out of place or some other punctuation.
- Logic error- When all the rules of the language have been followed but the programmer has made some other mistake that prevents the program from running as expected.
Types of test data
Test data can be normal, boundary or invalid / erroneous.
Let’s say we are testing the validation of a student’s test score. It should be between 1 and 100.
- Normal: some data that would normally be expected to be ok e.g. 56
- Boundary: data that is on the very edge of what should be expected, both inside and outside the bounds e.g. 0, 1, 100, 101
- Invalid: data that should not be accepted e.g. -10, £FISH^HVC