Component 2
2.2 Additional Programming Techniques
- String manipulation
- File handling
- Record data structure
- SQL
- Arrays
- Sub-programs
- Random number generation
String manipulation
Get the length of a string.
EXAM REFERENCE LANGUAGE |
name = "Frederick"
print(name.length) |
PYTHON |
name = "Frederick"
print(len(name)) |
C# |
string name = "Frederick";
Console.WriteLine(name.Length); |
Get part of a string. This example will print "Fred".
EXAM REFERENCE LANGUAGE |
name = "Frederick"
print(name.substring(0, 4)) |
PYTHON |
name = "Frederick"
print(name[0:4]) |
C# |
string name = "Frederick";
Console.WriteLine(name.Substring(0, 4)); |
Convert to upper case and lower case letters. Useful for validations.
EXAM REFERENCE LANGUAGE |
name = "Frederick"
print(name.upper)
print(name.lower) |
PYTHON |
name = "Frederick"
print(name.upper())
print(name.lower()) |
C# |
string name = "Frederick";
Console.WriteLine(name.ToUpper());
Console.WriteLine(name.ToLower()); |
Convert to ASCII and back again.
EXAM REFERENCE LANGUAGE |
print(ASC("A"))
print(CHR(65)) |
PYTHON |
print(ord("A"))
print(chr(65)) |
C# |
Console.WriteLine(Comvert.ToInt32('A'));
Console.WriteLine(Convert.ToChar(65)); |
File handling
Remember when using files you have to open them in either read, write or append mode.
You have to open the file, do your reading or writing, then close the file.
The following examples will read every line from a file and write a line to a file.
EXAM REFERENCE LANGUAGE |
myFile = openRead("story.txt")
while NOT myFile.endOfFile()
print(myFile.readLine())
endwhile
myFile.close()
myFile = openWrite("story.txt")
myFile.writeLine("The cat sat on the mat.")
myFile.close() |
PYTHON |
file = open("story.txt", "r")
for line in file:
print(line)
file.close()
file = open("story.txt", "w")
file.write("The cat sat on the mat.")
file.close() |
C# |
using (StreamReader reader = new StreamReader("story.txt")) {
while(!reader.EndOfStream) {
Console.WriteLine(reader.ReadLine());
}
}
using(StreamWriter writer = nwe StreamWriter("story.txt") {
writer.WriteLine("The cat sat on the mat.");
} |
Record data structure
A record data structure is a data structure that allows combining different data types into a single entity. It consists of multiple fields or members, where each field can store data of a different type. These fields are grouped together to form a record.
The implementation of records is a bit tricky. There is no reference to it in the exam board's guide to the exam reference language. In Python, the closest thing is called a dictionary. In C#, it is called a struct.
EXAM REFERENCE LANGUAGE |
//No reference to it in the guide. Expect to see it explained if it appears in an exam. |
PYTHON |
cat = {
"name" : "Felix",
"colour" : "Black",
"number_of_lives" : 9,
"isMale" : True
} |
C# |
struct Cat {
public string name;
public string colour;
public int numberOfLives;
public bool isMale;
}
Cat c = new Cat();
c.name = "Felix";
c.colour = "Black";
c.numberOfLives 9;
c.isMale = true; |
SQL
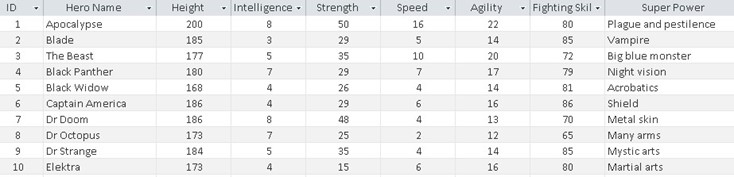
SQL is used to create and search databases. At GCSE level you only need to know how to search. You might be given a data table like the one above and be expected to state the results of some SQL queries.
Wildcards: * can be used to mean all fields should be returned or it could mean insert any number of letters.
% means insert any one letter.
LIKE: can be used to find values that are similar to the one in the statement.
SQL Query | Value returned |
---|---|
SELECT Strength FROM Heroes WHERE Hero Name = "Apocalypse" | 50 |
SELECT * FROM Heroes WHERE Strength > 40 | Gives all the fields from Apocalypse and Dr Doom |
SELECT Hero Name FROM Heroes WHERE Intelligence > 6 AND Speed > 7 | Apocalypse |
SELECT Hero Name FROM Heroes WHERE Intelligence > 6 OR Speed > 7 | Apocalypse, Black Panther, Dr Doom, Dr Octopus, The Beast |
Arrays
An array is a series of storage locations in memory that are named under one identifier. Each location is given an index beginning with zero.
Those of you who have studied Python should know that arrays do not exist in this language. Python uses lists instead which are similar. At GCSE level it is ok to assume that lists and arrays are the same thing.
Arrays can be one-dimensional (one row) or two-dimensional (many rows like a grid).
1D arrays

The following examples show how to declare and assign the array above. It then shows how to iterate through the array and print out each value.
EXAM REFERENCE LANGUAGE |
array animals[5]
animals[0] = "Dog"
animals[1] = "Horse"
animals[2] = "Fish"
animals[3] = "Cow"
animals[4] = "Sheep"
for i = 0 to animals.length
print(animals[i])
next i |
PYTHON |
animals = []
animals.append("Dog")
animals.append("Horse")
animals.append("Fish")
animals.append("Cow")
animals.append("Sheep")
for i in range(0, 5):
print(animals[i]) |
C# |
string[] animals = new string[5];
animals[0] = "Dog";
animals[1] = "Horse";
animals[2] = "Fish";
animals[3] = "Cow";
animals[4] = "Sheep";
for(int i = 0; i < animals.length; i++) {
Console.WriteLine(animals[i]);
} |
2D arrays
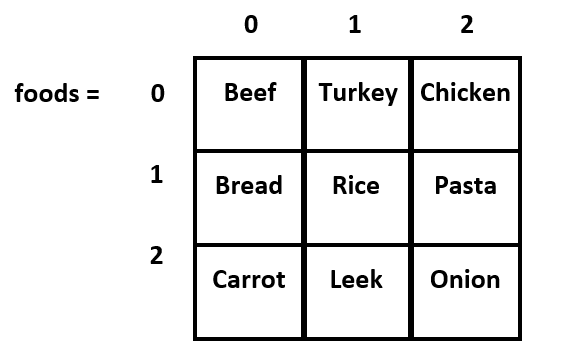
The following examples show how to declare and assign the 2D array above. Notice the indexes start in the top left! It then shows how to iterate through the array and print out each value. If using Python then technically you are creating a list of lists but don’t worry about it at GCSE level.
EXAM REFERENCE LANGUAGE |
array foods[3,3]
foods[0,0] = "Beef"
foods[0,1] = "Turkey"
foods[0,2] = "Chicken"
foods[1,0] = "Bread"
foods[1,1] = "Rice"
foods[1,2] = "Pasta"
foods[2,0] = "Carrot"
foods[2,1] = "Leek"
foods[2,2] = "Onion"
for i = 0 to 2
for j = 0 to 2
print(foods[i,j])
next j
next i |
PYTHON |
foods = []
foods.append(["Beef", "Turkey", "Chicken"])
foods.append(["Bread", "Rice", "Pasta"])
foods.append(["Carrot", "Leek", "Onion"])
for i in range(0, 3):
for j in range(0, 3):
print(foods[i][j]) |
C# |
string foods[,] = new string[3,3];
foods[0,0] = "Beef";
foods[0,1] = "Turkey";
foods[0,2] = "Chicken";
foods[1,0] = "Bread";
foods[1,1] = "Rice";
foods[1,2] = "Pasta";
foods[2,0] = "Carrot";
foods[2,1] = "Leek";
foods[2,2] = "Onion";
for(int i = 0; i < 3; i++) {
for(int j = 0; j < 3; j++) {
Console.WriteLine(foods[i, j]);
}
} |
Sub-programs
Sub-programs are named blocks of code that can be called and used over and over again. Using sub-programs helps to make your code efficient by avoiding repeating lines of code. The sub-programs can be reused saving development time. There are two types:
- Procedures- don't return a value.
- Functions- return a value.
Procedures
EXAM REFERENCE LANGUAGE |
procedure hello(name)
print("Hello " + name)
end procedure |
PYTHON |
def hello(name):
print("Hello " + name) |
C# |
static void Hello(string name) {
Console.WriteLine("Hello " + name);
} |
Functions
EXAM REFERENCE LANGUAGE |
function doubler(number)
return number * 2
end function |
PYTHON |
def doubler(number):
return number * 2 |
C# |
static decimal Doubler(decimal number) {
return number * 2;
} |
Random number generation
All languages have a routine or library capable of generating random numbers. These can be useful for many different reasons including making games e.g. dice rolls. These examples will display a random number between 1 and 10.
EXAM REFERENCE LANGUAGE |
r = random(1, 10)
print(r) |
PYTHON |
import random
r = random.randint(1, 11)
print(r) |
C# |
Random rnd = new Random();
int r = rnd.Next(1,11);
Console.WriteLine(r); |