Component 2
2.1 Computational Thinking
- Abstraction
- Decomposition
- Algorithmic thinking
- Inputs, processes and outputs
- Structure diagrams
- Flowcharts
- Pseudocode
- Exam Reference Language
- Identify common errors
- Trace tables
Abstraction
Making a problem simpler by removing unnecessary details. This is important when coding as it:
- Makes it easier to code!
- Means less time is wasted on the unnecessary details.
- Means the final product will use less memory and processor power.
e.g. we are making a system to track a pupil’s attendance in school:
Details we might leave in | Unecessary details we would leave out |
---|---|
Pupil names | Pupil eye colour |
Whether they were in or not on a certain day | Medical records |
Days of the week | Behaviour points |
Holidays | Phone number |
Decomposition
Breaking a difficult problem down into smaller and smaller sub problems until they are easy to solve. We use this in programming to break a project down until we can code each section with one function. This stops a programmer being overwhelmed by the thought of a huge task and allows them to focus on smaller, easier tasks that all connect together to create a full solution.
Algorithmic thinking
The spec says you need to think in algorithms… don’t worry! You have been learning this since you started to learn how to code. You will need to be able to understand and write flow diagrams and pseudocode.
Inputs, processes and outputs
All computer systems follow this format. Some data is inputted. This could be a mouse click, text entry, game controller or many other things.All The computer then does some processing. Finally, the results are outputted e.g. to a screen or with sound.
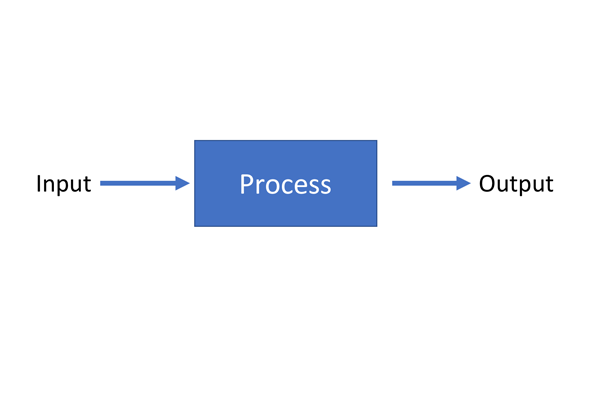
Structure diagram
These diagrams can be used when decomposing a problem. The idea is a main idea is at the top, and further sub-ideas are arranged on each level.x Here is an example for an endless runner style game.
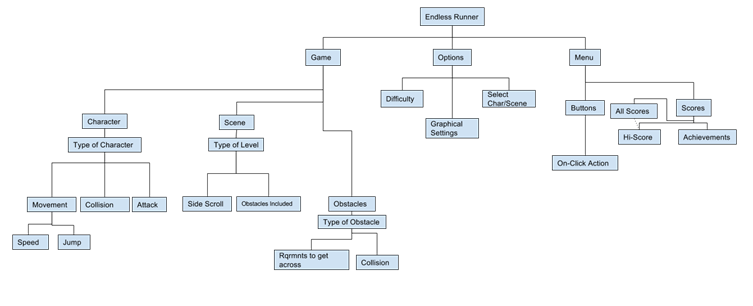
Flowcharts
When working with flowcharts, it is important to know the correct symbols. The ones you need to know are in the picture here.
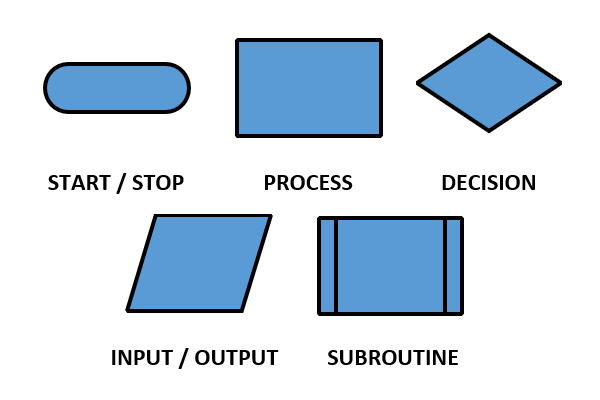
Pseudocode
The good news is that when writing pseudocode there isn’t a right or wrong. You can write anything that looks a bit like code and a bit like English. However, you do need to show the processes you would use when you write actual code. e.g. if you would use actual for loop to solve a problem, make sure there is something that looks reasonably like a for loop in your pseudocode.
Exam Reference Language
Writing a qualification for computer science is challenging as different learners like to use different programming languages. It wouldn't be fair for the exam board to feature any one programming language in the exam as it would exclude everybody who studied a different one!
To avoid this difficulty, the exam board have created a special Exam Reference Language that contains the most common features of all the major languages that can be studied for the course. You should become familiar with it as any code question is likely to feature it.
You can find a guide for Exam Reference language in the specification.
Common errors
You will be expected to look at code and be able to identify mistakes that would cause the code to not run properly. This is a skill that you can only gain through practical programming experience. The best advice is to practice programming regularly. Find something that inspires you e.g. make a website about a hobby or code a game. You will quickly make progress and improve your level whilst having fun at the same time.
Trace tables
A trace table is a table used to show all the steps of a particular algorithm. It is sometimes known as a dry run.
When writing out a trace table, you should write the line number and the value that a variable changes to or a vaue that is outputted. If a variables does not change value, there is no need to write it down.
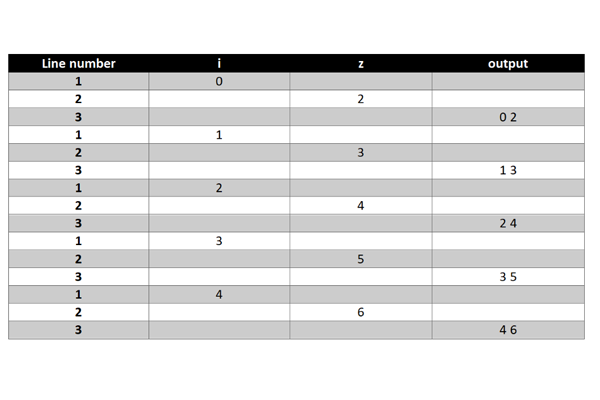